App Partner
Become an ActiveCampaign App Partner
Help over 180,000 businesses around the world deliver unique customer experiences by developing an app with ActiveCampaign.
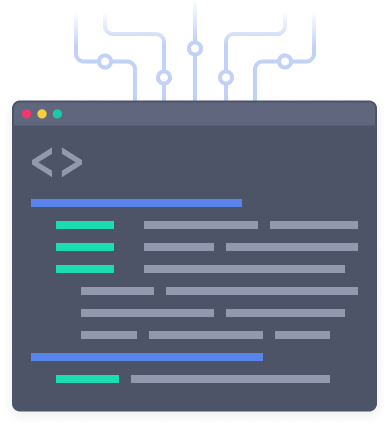
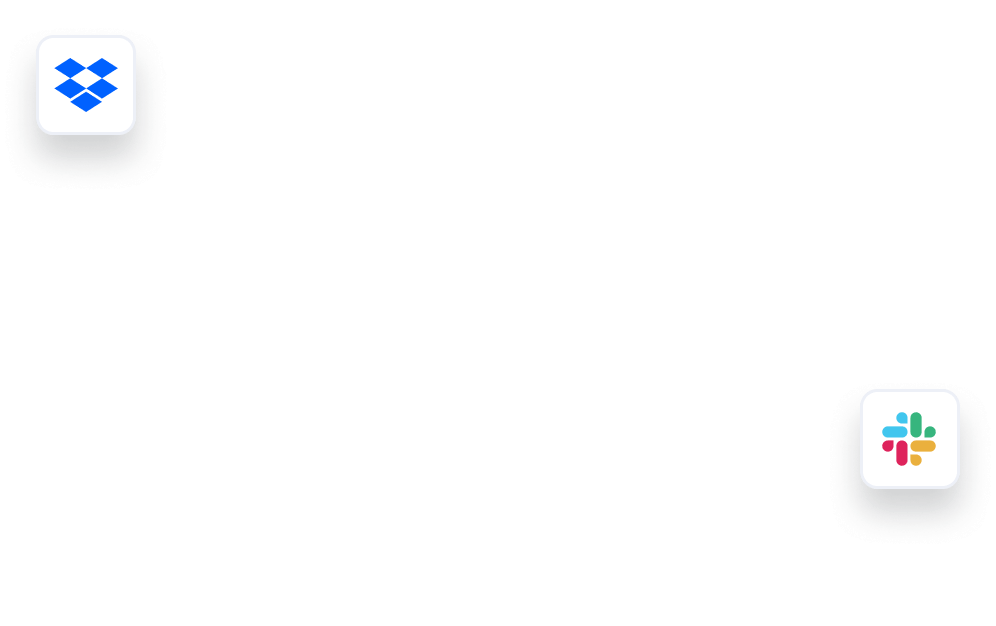
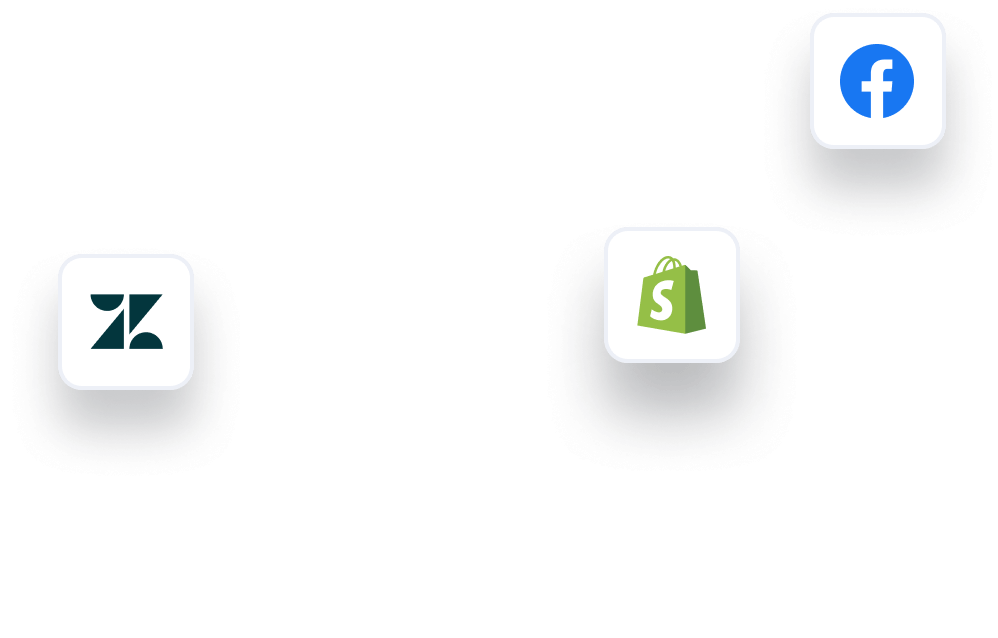
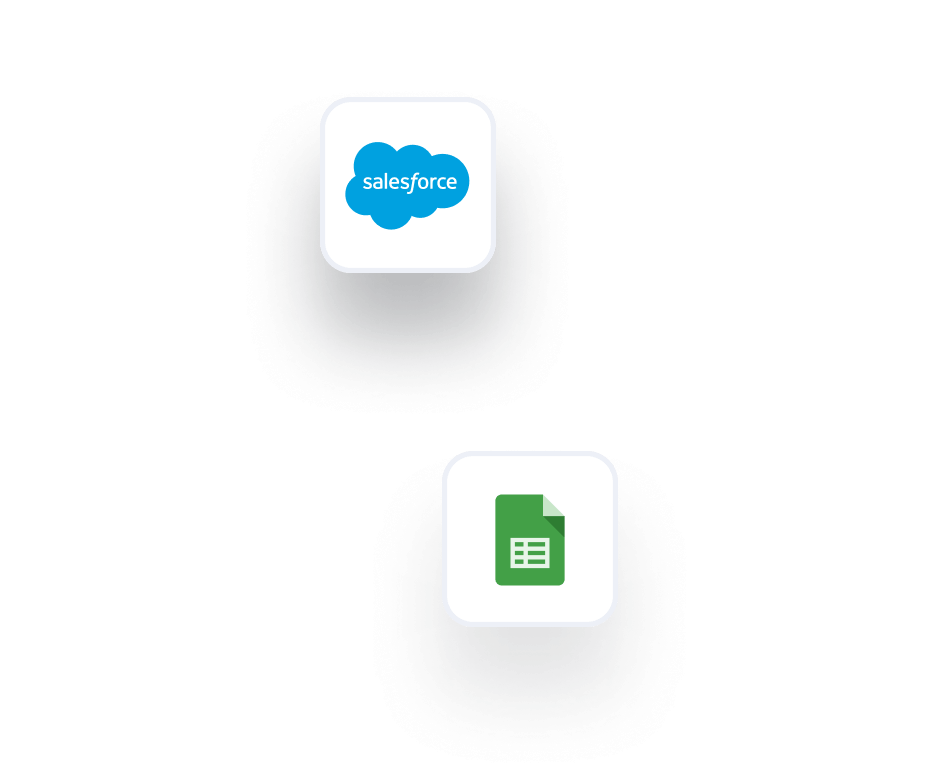
API Documentation
Version 3 of the ActiveCampaign API is structured around REST, HTTP, and JSON. API endpoint URLs are organized around resources, such as contacts or deals. That means you can easily call on ActiveCampaign actions for your integration.
Get API docs
App Studio
Build upon the leading Customer Experience Automation platform and expose your app to a growing ecosystem, and 180K+ customers globally.
Start building an app
Webhooks
Get notified at the URL of your choice when your contacts do something you care about—like opening a campaign or clicking a link.
Set up a webhook
Get started developing with ActiveCampaign in minutes
# Retrieve a contact
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://youraccountname.api-us1.com/api/3/contacts/id")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
response = http.request(request)
puts response.read_body
# Retrieve a contact
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://youraccountname.api-us1.com/api/3/contacts/id")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
response = http.request(request)
puts response.read_body
// Retrieve a contact
var request = require("request");
var options = {
method: 'GET',
url: 'https://youraccountname.api-us1.com/api/3/contacts/id'
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
// Retrieve a contact
var request = require("request");
var options = {
method: 'GET',
url: 'https://youraccountname.api-us1.com/api/3/contacts/id'
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
# Retrieve a contact
import requests
url = "https://youraccountname.api-us1.com/api/3/contacts/id"
response = requests.request("GET", url)
print(response.text)
# Retrieve a contact
import requests
url = "https://youraccountname.api-us1.com/api/3/contacts/id"
response = requests.request("GET", url)
print(response.text)
// Retrieve a contact
var data = null;
var xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://youraccountname.api-us1.com/api/3/contacts/id");
xhr.send(data);
// Retrieve a contact
var data = null;
var xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://youraccountname.api-us1.com/api/3/contacts/id");
xhr.send(data);
# Retrieve a contact
curl --request GET \
--url 'https://youraccountname.api-us1.com/api/3/contacts/id'
# Retrieve a contact
curl --request GET \
--url 'https://youraccountname.api-us1.com/api/3/contacts/id'
# Retrieve an account
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://youraccountname.api-us1.com/api/3/accounts/id")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
response = http.request(request)
puts response.read_body
# Retrieve an account
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://youraccountname.api-us1.com/api/3/accounts/id")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
response = http.request(request)
puts response.read_body
// Retrieve an account
var request = require("request");
var options = {
method: 'GET',
url: 'https://youraccountname.api-us1.com/api/3/accounts/id'
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
// Retrieve an account
var request = require("request");
var options = {
method: 'GET',
url: 'https://youraccountname.api-us1.com/api/3/accounts/id'
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
# Retrieve an account
import requests
url = "https://youraccountname.api-us1.com/api/3/accounts/id"
response = requests.request("GET", url)
print(response.text)
# Retrieve an account
import requests
url = "https://youraccountname.api-us1.com/api/3/accounts/id"
response = requests.request("GET", url)
print(response.text)
// Retrieve an account
var data = null;
var xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://youraccountname.api-us1.com/api/3/accounts/id");
xhr.send(data);
// Retrieve an account
var data = null;
var xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://youraccountname.api-us1.com/api/3/accounts/id");
xhr.send(data);
# Retrieve an account
curl --request GET \
--url 'https://youraccountname.api-us1.com/api/3/accounts/id'
# Retrieve an account
curl --request GET \
--url 'https://youraccountname.api-us1.com/api/3/accounts/id'
# Retrieve a deal
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://youraccountname.api-us1.com/api/3/deals/id")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
response = http.request(request)
puts response.read_body
# Retrieve a deal
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://youraccountname.api-us1.com/api/3/deals/id")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
response = http.request(request)
puts response.read_body
// Retrieve a deal
var request = require("request");
var options = {
method: 'GET',
url: 'https://youraccountname.api-us1.com/api/3/deals/id'
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
// Retrieve a deal
var request = require("request");
var options = {
method: 'GET',
url: 'https://youraccountname.api-us1.com/api/3/deals/id'
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
# Retrieve a deal
import requests
url = "https://youraccountname.api-us1.com/api/3/deals/id"
response = requests.request("GET", url)
print(response.text)
# Retrieve a deal
import requests
url = "https://youraccountname.api-us1.com/api/3/deals/id"
response = requests.request("GET", url)
print(response.text)
// Retrieve a deal
var data = null;
var xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://youraccountname.api-us1.com/api/3/deals/id");
xhr.send(data);
// Retrieve a deal
var data = null;
var xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://youraccountname.api-us1.com/api/3/deals/id");
xhr.send(data);
# Retrieve a deal
curl --request GET \
--url 'https://youraccountname.api-us1.com/api/3/deals/id'
# Retrieve a deal
curl --request GET \
--url 'https://youraccountname.api-us1.com/api/3/deals/id'
# Retrieve a campaign
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://youraccountname.api-us1.com/api/3/campaigns?orders%5Bsdate%5D=ASC")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
response = http.request(request)
puts response.read_body
# Retrieve a campaign
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://youraccountname.api-us1.com/api/3/campaigns?orders%5Bsdate%5D=ASC")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
response = http.request(request)
puts response.read_body
// Retrieve a campaign
var request = require("request");
var options = {
method: 'GET',
url: 'https://youraccountname.api-us1.com/api/3/campaigns',
qs: {'orders[sdate]': 'ASC'}
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
// Retrieve a campaign
var request = require("request");
var options = {
method: 'GET',
url: 'https://youraccountname.api-us1.com/api/3/campaigns',
qs: {'orders[sdate]': 'ASC'}
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
# Retrieve a campaign
import requests
url = "https://youraccountname.api-us1.com/api/3/campaigns"
querystring = {"orders[sdate]":"ASC"}
response = requests.request("GET", url, params=querystring)
print(response.text)
# Retrieve a campaign
import requests
url = "https://youraccountname.api-us1.com/api/3/campaigns"
querystring = {"orders[sdate]":"ASC"}
response = requests.request("GET", url, params=querystring)
print(response.text)
// Retrieve a campaign
var data = null;
var xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://youraccountname.api-us1.com/api/3/campaigns?orders%5Bsdate%5D=ASC");
xhr.send(data);
// Retrieve a campaign
var data = null;
var xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://youraccountname.api-us1.com/api/3/campaigns?orders%5Bsdate%5D=ASC");
xhr.send(data);
# Retrieve a campaign
curl --request GET \
--url 'https://youraccountname.api-us1.com/api/3/campaigns?orders%5Bsdate%5D=ASC'
# Retrieve a campaign
curl --request GET \
--url 'https://youraccountname.api-us1.com/api/3/campaigns?orders%5Bsdate%5D=ASC'
# Retrieve an automation
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://youraccountname.api-us1.com/api/3/automations")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
response = http.request(request)
puts response.read_body
# Retrieve an automation
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://youraccountname.api-us1.com/api/3/automations")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
response = http.request(request)
puts response.read_body
// Retrieve an automation
var request = require("request");
var options = {
method: 'GET',
url: 'https://youraccountname.api-us1.com/api/3/automations'
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
// Retrieve an automation
var request = require("request");
var options = {
method: 'GET',
url: 'https://youraccountname.api-us1.com/api/3/automations'
};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
# Retrieve an automation
import requests
url = "https://youraccountname.api-us1.com/api/3/automations"
response = requests.request("GET", url)
print(response.text)
# Retrieve an automation
import requests
url = "https://youraccountname.api-us1.com/api/3/automations"
response = requests.request("GET", url)
print(response.text)
// Retrieve an automation
var data = null;
var xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://youraccountname.api-us1.com/api/3/automations");
xhr.send(data);
// Retrieve an automation
var data = null;
var xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://youraccountname.api-us1.com/api/3/automations");
xhr.send(data);
# Retrieve an automation
curl --request GET \
--url 'https://youraccountname.api-us1.com/api/3/automations'
# Retrieve an automation
curl --request GET \
--url 'https://youraccountname.api-us1.com/api/3/automations'
App Studio
See what’s possible with the new ActiveCampaign App Studio
Easy access
Access App Studio from any ActiveCampaign account, including developer sandbox accounts.
Edit live
Use the live editor with in-line validations to quickly build your app.
Test your app
Easily test your app with live logs to help you debug along the way.
Manage versions
With version management you can save, exit and return to your in-progress app at any time.
Publish to the Ecosystem
Publish your app to our ecosystem of 350+ apps and gain exposure to over 180K+ customers around the globe.





App Marketplace
Reach 180,000+ potential customers
Share your app with 180K+ customers in an App Marketplace full of hundreds of integrations for all types of businesses.
Explore the App Marketplace Already have an app? Get it listed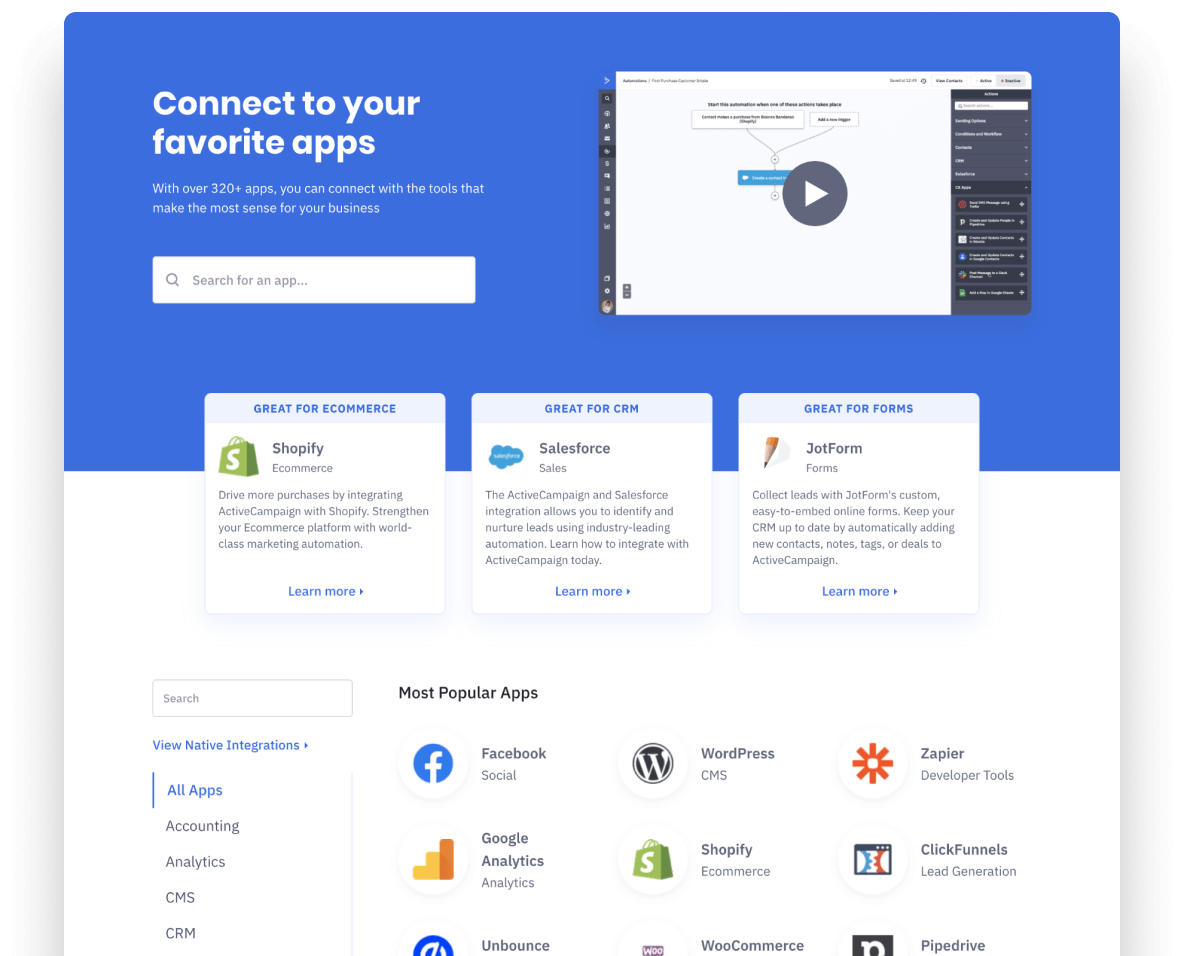
OUT-OF-THE BOX DOESN'T WORK FOR EVERY BUSINESS
Build unique solutions for unique businesses
Payment driven workflows to drive referrals and customer loyalty
Automate appointment reminders with SMS
Manage multiple lead channels with our Deals CRM
Improve every customer experience via powerful feedback-based marketing automations
Drive more donations with personalization and eCommerce reporting, attribution and segmentation
Centralize unsubscribes and sync other databases automatically






WHY DEVELOPERS CHOOSE ACTIVECAMPAIGN
Hear what other developers are saying about partnering with ActiveCampaign
70% of ActiveCampaign’s 180,000+ customers use an integration. It could be yours.
0
customers world wide
A global footprint
ActiveCampaign has a global network of 180K+ customers in over 170 countries
0
use an integration
Highly engaged customers
The vast majority of our customers are utilizing integrations to customize the platform in a way that makes sense for their business
Highest Rated
in Marketing Automation
An industry leader
Our category-defining Customer Experience Automation platform is one of the top 50 Marketing Products according to G2
Start building with ActiveCampaign today
Become an App Partner and start creating unique customer experiences